Basic 1
Overview
This example will display a dual multi-select QuickForm element, to manage
a simple car list.
Only default options are used.
Screenshot
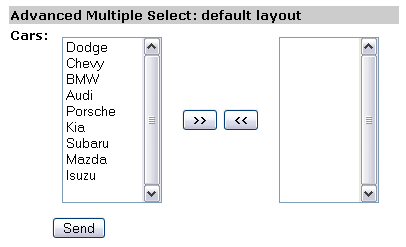
Demonstration
Give it a try
Dependencies
This example requires mandatory resources :
Explains step by step
Step 1
First, we loads the class definitions for QuickForm and advmultiselect element
(lines 17,18), then we creates a HTML_QuickForm object
that will contain the object representing elements and all the other necessary information.
We only pass the form's name to the constructor, which means that default values
will be used for other parameters (line 20).
The dual multi-select is created (line 55) with
no headers except global element title 'Cars:' and load with data defined from $car_array
(lines 40 thru 50).
Both list are 100 pixels width and display 10 items:
Property | Value | Default |
size | | 10 |
width | | 100 |
class | | |
Step 2
The basic render is built with default element template and default class declarations:
none means (table border=0 cellpadding=10 cellspacing=0).
Here is the default element template:
{javascript}
<table{class}>
<!-- BEGIN label_2 --><tr><th>{label_2}</th><!-- END label_2 -->
<!-- BEGIN label_3 --><th> </th><th>{label_3}</th></tr><!-- END label_3 -->
<tr>
<td valign="top">{unselected}</td>
<td align="center">{add}{remove}</td>
<td valign="top">{selected}</td>
</tr>
</table>
The swap buttons used defaults that may be retrieve if you don't specify attributes
on setButtonAttributes() method.
Property | Value | Default |
name | | add |
value | | >> |
type | | button |
Property | Value | Default |
name | | remove |
value | | << |
type | | button |
Step 3
Immediately before the form is displayed (line 84)
we attempt to validate it (line 77).
Results of car selection are displayed on lines lines 78 to 82.
Source Code
PHP code
<?php
/**
* Basic advMultiSelect HTML_QuickForm element
* without any customization.
*
* @version $Id: qfams_basic_1.php,v 1.5 2009/01/28 22:24:43 farell Exp $
* @author Laurent Laville <pear@laurent-laville.org>
* @package HTML_QuickForm_advmultiselect
* @subpackage Examples
* @access public
* @example examples/qfams_basic_1.php
* qfams_basic_1 source code
* @link http://www.laurent-laville.org/img/qfams/screenshot/basic1.png
* screenshot (Image PNG, 406x247 pixels) 4.95 Kb
*/
require_once 'HTML/QuickForm.php';
require_once 'HTML/QuickForm/advmultiselect.php';
$form = new HTML_QuickForm('amsBasic1');
$form->removeAttribute('name'); // XHTML compliance
// same as default element template but wihtout the label (in first td cell)
$withoutLabel = <<<_HTML
<tr valign="top">
<td align="right">
</td>
<td align="left">
<!-- BEGIN error --><span style="color: #ff0000;">{error}</span><br /><!-- END error -->{element}
</td>
</tr>
_HTML;
// more XHTML compliant
// replace default element template with label, because submit button have no label
$renderer =& $form->defaultRenderer();
$renderer->setElementTemplate($withoutLabel, 'send');
$car_array = array(
'dodge' => 'Dodge',
'chevy' => 'Chevy',
'bmw' => 'BMW',
'audi' => 'Audi',
'porsche' => 'Porsche',
'kia' => 'Kia',
'subaru' => 'Subaru',
'mazda' => 'Mazda',
'isuzu' => 'Isuzu',
);
// rendering with all default options
$form->addElement('header', null, 'Advanced Multiple Select: default layout ');
$form->addElement('advmultiselect', 'cars', 'Cars:', $car_array);
$form->addElement('submit', 'send', 'Send');
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN"
"http://www.w3c.org/TR/xhtml1/DTD/xhtml1-strict.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" />
<title>HTML_QuickForm::advMultiSelect basic example 1</title>
<style type="text/css">
<!--
body {
background-color: #FFF;
font-family: Verdana, Arial, helvetica;
font-size: 10pt;
}
-->
</style>
</head>
<body>
<?php
if ($form->validate()) {
$clean = $form->getSubmitValues();
echo '<pre>';
print_r($clean);
echo '</pre>';
}
$form->display();
?>
</body>
</html>