Custom Monitor
Overview
This example will run a Progress2 Monitor in indeterminate mode.
The progress bar starts in indeterminate mode then switch back and finish in determinate mode.
You don't need to click on Start button to begin, due to autorun option set to true
(see class constructor).
Screenshot
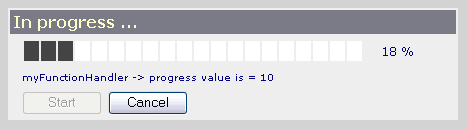
Demonstration
Give it a try
Dependencies
This example requires mandatory resources :
And also but optional :
Explains step by step
The progress meter wait 100ms (line 49) between each step of 1% (default).
The form windows (frmMonitor4) have default title (In progress ...),
buttons name (Start, Cancel) with size set to 80 pixels width,
and autorun option set to true (line 44) :
see class constructor parameters (lines 42-46).
There are 20 cells (line 50) 15x20:
Property | Value | Default |
active-color | #444444 | #006600 |
inactive-color | #FFF | #CCCCCC |
width | | 15 |
height | | 20 |
spacing | | 2 |
See also :
With percent text info aligned on right side:
Property | Value | Default |
left | | 5 |
top | | 5 |
width | | 50 |
height | | 0 |
align | | right |
valign | | right |
background-color | | |
font-size | | 11 |
font-family | | Verdana, Tahoma, Arial |
font-weight | | normal |
color | navy | #000000 |
class | | progressPercentLabel%s |
And the monitor status line (default monitorStatus) aligned at bottom of progress bar
(line 54) with:
Property | Value | Default |
left | | 5 |
top | | 5 |
width | | 50 |
height | | 0 |
align | | right |
valign | | right |
background-color | | |
font-size | 10 | 11 |
font-family | | Verdana, Tahoma, Arial |
font-weight | | normal |
color | navy | #000000 |
class | | progressPercentLabel%s |
See also :
QF renderer is defined on lines 79-100.
Source Code
<?php
/**
* Progress2 Monitor
* with a new form template and progress bar color layout.
* Used a function as user callback.
*
* @version $Id: monitorcus.php,v 1.4 2006/05/24 08:42:43 farell Exp $
* @author Laurent Laville <pear@laurent-laville.org>
* @package HTML_Progress2
* @subpackage Examples
* @access public
*/
require_once 'HTML/Progress2/Monitor.php';
/**
* In case we have attached an indeterminate progress bar to the monitor
* once we have reached 60%,
* we swap from indeterminate mode to determinate mode
* and run a standard progress bar from 0 to 100%
*
* @param int $pValue current value of the progress bar
* @param object $pMon the progress monitor itself
*/
function myFunctionHandler($pValue, &$pb)
{
global $pm;
$pb->sleep();
if (!$pb->isIndeterminate()) {
if (fmod($pValue,10) == 0) {
$pm->setCaption('myFunctionHandler -> progress value is = %value%',
array('value' => $pValue)
);
}
} elseif ($pValue == 60) {
$pb->setIndeterminate(false);
$pb->setValue(0);
}
}
$pm = new HTML_Progress2_Monitor('frmMonitor4', array(
'button' => array('style' => 'width:80px;'),
'autorun' => true
)
);
$pb =& $pm->getProgressElement();
$pb->setAnimSpeed(100);
$pb->setCellCount(20);
$pb->setProgressAttributes('background-color=#EEE');
$pb->setCellAttributes('inactive-color=#FFF active-color=#444444');
$pb->setLabelAttributes('pct1', 'color=navy');
$pb->setLabelAttributes('monitorStatus', 'color=navy font-size=10');
$pb->setIndeterminate(true);
$pb->setProgressHandler('myFunctionHandler');
$pm->setProgressElement($pb);
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en">
<head>
<title>Custom Progress2 Monitor </title>
<style type="text/css">
<!--
body {
background-color: lightgrey;
font-family: Verdana, Arial;
}
<?php echo $pm->getStyle(); ?>
-->
</style>
<?php echo $pm->getScript(false); ?>
</head>
<body>
<?php
$renderer =& HTML_QuickForm::defaultRenderer();
$renderer->setFormTemplate('
<form{attributes}>
<table width="450" border="0" cellpadding="3" cellspacing="2" bgcolor="#EEEEEE">
{content}
</table>
</form>
');
$renderer->setElementTemplate('
<tr>
<td valign="top" style="padding-left:15px;">
{element}
</td>
</tr>
');
$renderer->setHeaderTemplate('
<tr>
<td style="background:#7B7B88;color:#ffc;" align="left" colspan="2">
<b>{header}</b>
</td>
</tr>
');
$pm->accept($renderer);
echo $renderer->toHtml();
$pm->run();
?>
</body>
</html>