IT dynamic renderer
Overview
This example will run a basic Progress2 Monitor, which used a IT dynamic
QuickForm renderer.
The process is a picture file upload simulation.
Screenshot
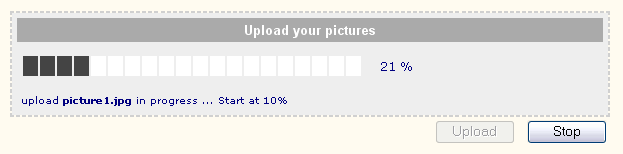
Demonstration
Give it a try
Dependencies
This example requires mandatory resources :
And also but optional :
Explains step by step
The progress meter wait 50ms (line 53) between each step of 1% (default).
The form windows (frmMonitor5) have title (Upload your pictures),
buttons name (Upload, Stop) with size set to 80 pixels width :
see class constructor parameters (lines 45-50).
There are 20 cells (line 54) 15x20 line 56:
Property | Value | Default |
active-color | #444444 | #006600 |
inactive-color | #FFF | #CCCCCC |
width | | 15 |
height | | 20 |
spacing | | 2 |
See also :
With percent text info aligned on right side:
Property | Value | Default |
left | | 5 |
top | | 5 |
width | | 50 |
height | | 0 |
align | | right |
valign | | right |
background-color | | |
font-size | | 11 |
font-family | | Verdana, Tahoma, Arial |
font-weight | | normal |
color | navy | #000000 |
class | | progressPercentLabel%s |
And the monitor status line (default monitorStatus) aligned at bottom of progress bar
(line 58) with:
Property | Value | Default |
left | | 5 |
top | | 5 |
width | | 50 |
height | | 0 |
align | | right |
valign | | right |
background-color | | |
font-size | 10 | 11 |
font-family | | Verdana, Tahoma, Arial |
font-weight | | normal |
color | navy | #000000 |
class | | progressPercentLabel%s |
See also :
Source Code
<?php
/**
* Progress2 Monitor using ITDynamic QF renderer,
* and a class-method as user callback.
*
* @version $Id: monitordyn.php,v 1.2 2005/08/01 08:35:03 farell Exp $
* @author Laurent Laville <pear@laurent-laville.org>
* @package HTML_Progress2
* @subpackage Examples
* @access public
*/
require_once 'HTML/Progress2/Monitor.php';
require_once 'HTML/QuickForm/Renderer/ITDynamic.php';
require_once 'HTML/Template/Sigma.php';
class myClassHandler
{
function myMethod($pValue, &$pb)
{
global $pm;
switch ($pValue) {
case 10:
$pic = 'picture1.jpg';
break;
case 45:
$pic = 'picture2.jpg';
break;
case 70:
$pic = 'picture3.jpg';
break;
default:
$pic = null;
}
if (!is_null($pic)) {
$pm->setCaption('upload %file% in progress ... '
. 'Start at %percent%%',
array('file' => $pic, 'percent' => $pValue)
);
}
$pb->sleep();
}
}
$pm = new HTML_Progress2_Monitor('frmMonitor5', array(
'title' => 'Upload your pictures',
'start' => 'Upload',
'cancel' => 'Stop',
'button' => array('style' => 'width:80px;')
));
$pb =& $pm->getProgressElement();
$pb->setAnimSpeed(50);
$pb->setCellCount(20);
$pb->setProgressAttributes('background-color=#EEE');
$pb->setCellAttributes('inactive-color=#FFF active-color=#444444');
$pb->setLabelAttributes('pct1', 'color=navy');
$pb->setLabelAttributes('monitorStatus', 'color=navy font-size=10');
$pb->setProgressHandler(array('myClassHandler','myMethod'));
$pm->setProgressElement($pb);
$tpl =& new HTML_Template_Sigma('.');
$tpl->loadTemplateFile('itdynamic.html');
$tpl->setVariable(array(
'qf_style' => $pm->getStyle(),
'qf_script' => $pm->getScript(false)
)
);
$renderer =& new HTML_QuickForm_Renderer_ITDynamic($tpl);
$renderer->setElementBlock(array('buttons' => 'qf_buttons'));
$pm->accept($renderer);
$tpl->show();
$pm->run();
?>
</body>
</html>