BlueSand with AJAX
Overview
This example will run a natural horizontal progress bar with blue skin (example 1.3),
in asynchronous mode.
Use an auto (HTML_)AJAX server with PHP class and methods.
Screenshot
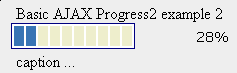
Demonstration
Give it a try
Dependencies
And also but optional :
Explains step by step
Lines 11-16:
clean-up session data when user-task is over (progress meter at 100%). The
default behavior of AJAX progress bar is to reload the same page when task is complete.
Use QueryString "reload=true". To change this behavior, you have to modify content of
HTML_Progress2.onComplete JavaScript variable (See example 15.4 with usage of Scriptaculous AJAX framework).
Lines 20-31, 39:
Reuse an existing external style sheet (see bluesand2.css)
following feature introduced in version 2.2.0
Take care to use the same progress bar identifier in your PHP (line 24)
and CSS code (lines 1,9,18,28,32)
Line 32:
function HTML_Progress2::registerAJAX, allow to identify components of (HTML_)AJAX
server. Here we don't use the default server name ('server.php')
but script 'auto_server.php' that will handle through PHP class RequestStatus (see line 51) and
one of its methods updatePercentage (see line 52) the simulation of a user-task that
take many cycles to complete, and return new status of the progress bar
(percentage and labels key-value pairs).
Line 47:
Do all job to initialize references to AJAX server and JS client librairies used.
Will print these lines:
<script type='text/javascript' src='auto_server.php?client=all'></script>
<script type='text/javascript' src='auto_server.php?stub=requeststatus'></script>
<script type="text/javascript">HTML_AJAX.defaultServerUrl = 'auto_server.php'</script>
Lines 51-52:
Identify the PHP class/method that will act as responder to handle user-task
(RequestStatus.class.php lines 90,100-105)
and update the progress bar status.
Source Code
default2.php
<?php
/**
* Basic AJAX example that used an auto server to handle progress bar responses
*
* @author Laurent Laville <pear@laurent-laville.org>
* @package HTML_Progress2
* @subpackage Examples
* @access public
*/
if (isset($_GET['reload'])) {
session_start();
unset($_SESSION['progressPercentage']);
session_destroy();
die('Job is over !');
}
require_once 'HTML/Progress2.php';
$pb = new HTML_Progress2();
$pb->addLabel(HTML_PROGRESS2_LABEL_TEXT, 'txt1', 'Basic AJAX Progress2 example 2');
$pb->addLabel(HTML_PROGRESS2_LABEL_TEXT, 'txt2', 'caption ...');
$pb->setLabelAttributes('txt2', array('valign' => 'bottom'));
$pb->setIdent('PB1');
$pb->setBorderAttributes('class=progressBorder');
$pb->setCellAttributes('class=cell');
$pb->setLabelAttributes('pct1', array(
'class' => 'progressPercentLabel',
'align' => 'center'
));
$pb->importStyle('bluesand2.css');
$pb->registerAJAX('auto_server.php', array('requeststatus'));
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN"
"http://www.w3c.org/TR/xhtml1/DTD/xhtml1-strict.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en">
<head>
<title>Basic Ajax Progress2 example 2</title>
<link rel="stylesheet" type="text/css" href="bluesand2.css" />
<style type="text/css">
<!--
body {background-color: #EEEEEE; }
-->
</style>
<script type="text/javascript" src="HTML_Progress2.js"></script>
<?php
echo $pb->setupAJAX();
?>
<script type="text/javascript">
//<![CDATA[
HTML_Progress2.serverClassName = 'RequestStatus';
HTML_Progress2.serverMethodName = 'updatePercentage';
//]]>
</script>
</head>
<body>
<a href="javascript:HTML_Progress2.start('<?php echo $pb->getIdent(); ?>');">Start</a>
<?php $pb->display(); ?>
</body>
</html>
auto_server.php
<?php
/**
* Auto server that will serve user task script responder
*
* @version $Id: auto_server.php,v 1.1 2007/01/22 17:58:54 farell Exp $
* @author Laurent Laville <pear@laurent-laville.org>
* @package HTML_Progress2
* @subpackage Examples
* @access public
*/
session_start();
require_once 'HTML/AJAX/Server.php';
class AutoServer extends HTML_AJAX_Server
{
// this flag must be set for your init methods to be used
var $initMethods = true;
function initRequestStatus()
{
include_once 'RequestStatus.class.php';
$status = new RequestStatus();
$this->registerClass($status, 'RequestStatus', array('updatePercentage'));
}
function initRequestFullStatus()
{
include_once 'RequestStatus.class.php';
$status = new RequestStatus();
$this->registerClass($status, 'RequestFullStatus', array('updateTask'));
}
function initRequestStatusAndFx()
{
include_once 'RequestStatus.class.php';
$status = new RequestStatus();
$this->registerClass($status, 'RequestStatusAndFx', array('updateTask'));
$this->registerJsLibrary('scriptaculous',
array('prototype.js', 'scriptaculous.js', 'effects.js'),
dirname(__FILE__) . DIRECTORY_SEPARATOR. 'scriptaculous-js-1.7.0' . DIRECTORY_SEPARATOR);
}
}
$server = new AutoServer();
$server->handleRequest();
?>
RequestStatus.class.php
<?php
/**
* Sample of user-task script, that also handle response for progress meter
*
* @version $Id: RequestStatus.class.php,v 1.1 2007/01/22 17:57:26 farell Exp $
* @author Laurent Laville <pear@laurent-laville.org>
* @package HTML_Progress2
* @subpackage Examples
* @access public
*/
class RequestStatus
{
var $status = array('labels' => array(), 'percentage' => 0);
function RequestStatus()
{
if (!isset($_SESSION['progressPercentage'])) {
$_SESSION['progressPercentage'] = 0;
}
}
/**
* Returns only new percentage value
*
* @return array
* @access public
*/
function getStatus()
{
return $this->status;
}
/**
* Returns new percentage value with associate new labels values.
*
* You can define label value even, if its not declared in progress bar;
* no error will be raised.
* See example default3.php and label id 'txt4' usage
*
* @return array
* @access public
*/
function getFullStatus()
{
$status = $this->getStatus();
if ($status['percentage'] < 25) {
$this->status['labels']['txt1'] = '1st quarter';
$this->status['labels']['txt2'] = 'Q1';
} elseif ($status['percentage'] < 50) {
$this->status['labels']['txt1'] = '2nd quarter';
$this->status['labels']['txt2'] = 'Q2';
} elseif ($status['percentage'] < 75) {
$this->status['labels']['txt1'] = '3rd quarter';
$this->status['labels']['txt2'] = 'Q3';
} else {
$this->status['labels']['txt1'] = '4th quarter';
$this->status['labels']['txt4'] = 'Q4';
}
return $this->status;
}
/**
* Move on current user-task and returns full status of progress meter.
*
* Here we simulate a user-task that take many cycles to complete, and we
* don't know how many.
*
* @return array
* @access public
*/
function updateTask()
{
$this->_updateStatus();
return $this->getFullStatus();
}
/**
* Move on current user-task and returns only new percentage of progress meter.
*
* Here we simulate a user-task that take many cycles to complete, and we
* don't know how many.
*
* @return array
* @access public
*/
function updatePercentage()
{
$this->_updateStatus();
return $this->getStatus();
}
/**
* Updates only progress meter status
*
* @return void
* @access private
*/
function _updateStatus()
{
$newVal = $_SESSION['progressPercentage'] + mt_rand(1, 25);
$_SESSION['progressPercentage'] = min(100, $newVal);
$this->status['percentage'] = $_SESSION['progressPercentage'];
}
}
?>
bluesand2.css
#PB1 .cellI, #PB1 .cellA {
width: 10px;
height: 20px;
font-family: Courier, Verdana;
font-size: 8px;
float: left;
}
#PB1 .progressBorder {
width: 122px;
height: 24px;
border-width: 1px;
border-style: solid;
border-color: navy;
background-color: #FFFFFF;
}
#PB1 .progressPercentLabel {
width: 60px;
text-align: center;
background-color: transparent;
font-size: 14px;
font-family: Verdana, Tahoma, Arial;
font-weight: normal;
color: #000000;
}
#PB1 .cellI {
background-color: #EEEECC;
}
#PB1 .cellA {
background-color: #3874B4;
}