Basic Indeterminate
Overview
This example will run a basic progress bar in indeterminate mode (without monitor)
Until it reaches a limit (elapse time > 12 seconds). Then the progress bar
will switch in determinate mode (default) and finish a full loop from 0 to 100%
by step +5.
Screenshot
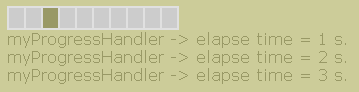
Demonstration
Give it a try
Dependencies
This example requires mandatory resources :
And also but optional :
Explains step by step
The progress meter wait 200ms (line 48)
between each step (line 49) in indeterminate mode.
This mode is activated on line 53.
Background of progress bar is changed (line 50):
Property | Value | Default |
class | | progressBar |
background-color | #E0E0E0 | #FFFFFF |
auto-size | | true |
See also :
There are 10 cells with :
Property | Value | Default |
active-color | #996 | #006600 |
inactive-color | | #CCCCCC |
width | | 15 |
height | | 20 |
spacing | | 2 |
See also :
And the percent text info (line 52) when progress meter will be in determinate mode:
Property | Value | Default |
id | | installationProgress |
width | | 50 |
font-family | | Verdana, Arial, Helvetica, sans-serif |
font-size | | 12 |
color | #996 | #000000 |
background-color | #CCCC99 | #FFFFFF |
align | | right |
valign | | right |
See also :
Source Code
<?php
/**
* Horizontal progress bar in indeterminate mode
* without using the Progress2_Monitor solution.
*
* @version $Id: half.php,v 1.4 2006/05/24 08:45:38 farell Exp $
* @author Laurent Laville <pear@laurent-laville.org>
* @package HTML_Progress2
* @subpackage Examples
* @access public
*/
require_once 'HTML/Progress2.php';
/**
* The progress bar will switch from indeterminate to determinate mode
* after a 12 seconds time elapsed.
*
* @param int $pValue current value of the progress bar
* @param object $pBar the progress bar itself
*/
function myProgressHandler($pValue, &$pBar)
{
static $c, $t;
if (!isset($c)) {
$c = time();
$t = 0;
}
$pBar->sleep();
if ($pBar->isIndeterminate()) {
$elapse = time() - $c;
if ($elapse > $t) {
echo "myProgressHandler -> elapse time = $elapse s.<br />\n";
$t++;
}
if ($elapse >= 12) {
$pBar->setIndeterminate(false);
$pBar->setValue(0);
$pBar->setIncrement(5);
}
}
}
$pb = new HTML_Progress2();
$pb->setAnimSpeed(200);
$pb->setIncrement(10);
$pb->setProgressAttributes('background-color=#E0E0E0');
$pb->setCellAttributes('active-color=#996');
$pb->setLabelAttributes('pct1', array('color' => '#996'));
$pb->setIndeterminate(true);
$pb->setProgressHandler('myProgressHandler');
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN"
"http://www.w3c.org/TR/xhtml1/DTD/xhtml1-strict.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en">
<head>
<title>Half Indeterminate Progress2 example</title>
<style type="text/css">
<!--
body {
background-color: #CCCC99;
color: #996;
font-family: Verdana, Arial;
}
<?php echo $pb->getStyle(); ?>
-->
</style>
<?php echo $pb->getScript(false); ?>
</head>
<body>
<?php
$pb->display();
echo '<br /><br />';
$pb->run();
?>
<p><b>Process Ended !</b></p>
</body>
</html>