Default observer
Overview
This example will run a progress bar with the default observer pattern
It will generates a storable representation of each progress bar changes.
Screenshot

And the file
progress_observer.log will contents:
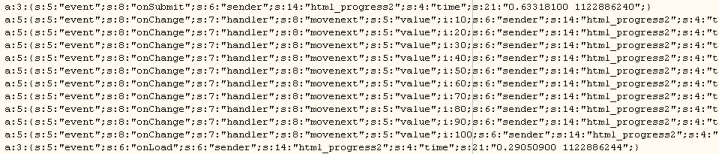
Demonstration
Give it a try
Dependencies
This example requires mandatory resources :
And also but optional :
Explains step by step
The progress meter wait 200ms (line 27)
between each step of 10% (line 28).
There are 10 cells with default values:
Property | Value | Default |
active-color | | #006600 |
inactive-color | | #CCCCCC |
width | | 15 |
height | | 20 |
spacing | | 2 |
See also :
Surrounded by a solid 2 pixels border (lines 35-36):
Property | Value | Default |
class | | progressBorder%s |
width | 2 | 0 |
style | | solid |
color | | #000000 |
See also :
Source Code
<?php
/**
* A basic observer for progress meter.
*
* @version $Id: observer1.php,v 1.4 2006/05/24 08:41:45 farell Exp $
* @author Laurent Laville <pear@laurent-laville.org>
* @package HTML_Progress2
* @subpackage Examples
* @access public
*/
require_once 'HTML/Progress2.php';
// Observer that record serialized event datas
function myObserver(&$notification)
{
$notifyName = $notification->getNotificationName();
$notifyInfo = $notification->getNotificationInfo();
$info = array_merge(array('event' => $notifyName), $notifyInfo);
$msg = serialize($info);
error_log ("$msg \n", 3, 'progress_observer.log');
}
// 1. Creates progress meter
$pb = new HTML_Progress2();
$pb->setComment('Basic Observer Progress2 example');
$pb->setAnimSpeed(200);
$pb->setIncrement(10);
// 2. Attach an observer
$pb->addListener('myObserver');
// 3. Changes look-and-feel of progress meter
// ( border: 2px, solid, #000000 )
$pb->setBorderPainted(true);
$pb->setBorderAttributes('width=2');
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN"
"http://www.w3c.org/TR/xhtml1/DTD/xhtml1-strict.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en">
<head>
<title>Default Observer Progress2 example</title>
<style type="text/css">
<!--
<?php echo $pb->getStyle(); ?>
body {
background-color: #FFFFFF;
}
-->
</style>
<?php echo $pb->getScript(false); ?>
</head>
<body>
<?php
$pb->display();
$pb->run();
?>
</body>
</html>